-
Notifications
You must be signed in to change notification settings - Fork 3.3k
/
README.md
443 lines (205 loc) · 8.32 KB
/
README.md
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
309
310
311
312
313
314
315
316
317
318
319
320
321
322
323
324
325
326
327
328
329
330
331
332
333
334
335
336
337
338
339
340
341
342
343
344
345
346
347
348
349
350
351
352
353
354
355
356
357
358
359
360
361
362
363
364
365
366
367
368
369
370
371
372
373
374
375
376
377
378
379
380
381
382
383
384
385
386
387
388
389
390
391
392
393
394
395
396
397
398
399
400
401
402
403
404
405
406
407
408
409
410
411
412
413
414
415
416
417
418
419
420
421
422
423
424
425
426
427
428
429
430
431
432
433
434
435
436
437
438
439
440
441
442
443
# Daily-Interview-Question
加入「前端面试互助群」学习小组,搜索公众号「高级前端进阶」,关注即可加入!
工作日每天一道大厂前端面试题,一年后再回头,会感谢曾经努力的自己!
[线上版本阅读更流畅,点击阅读](https://muyiy.cn/question/)
<br/>
[推荐一个不错的前端算法系列,点击查看](https://github.com/sisterAn/JavaScript-Algorithms)
<br/>
推荐扫码使用微信小程序,除了本项目之外,还囊括了算法题、选择题等多种类型题目和详细解析
记住我们的 Slogan:上下班路上刷一点,半年突击进大厂
<img src="http://resource.muyiy.cn/image/20200106214930.jpg" height="250px">
<br/>
## 今日面试题
第 162 题:实现对象的 Map 函数类似 Array.prototype.map
解析:[第 162 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/431)
<br/>
## 最近汇总
第 161 题:用最精炼的代码实现数组非零非负最小值 index
```js
// 例如:[10,21,0,-7,35,7,9,23,18] 输出 5, 7 最小
function getIndex(arr){
let index=null;
...
return index;
}
```
解析:[第 161 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/421)
<br/>
第 160 题:输出以下代码运行结果,为什么?如果希望每隔 1s 输出一个结果,应该如何改造?注意不可改动 square 方法
```js
const list = [1, 2, 3]
const square = num => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(num * num)
}, 1000)
})
}
function test() {
list.forEach(async x=> {
const res = await square(x)
console.log(res)
})
}
test()
```
解析:[第 160 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/389)
<br/>
第 159 题:实现 `Promise.retry`,成功后 `resolve` 结果,失败后重试,尝试超过一定次数才真正的 `reject`
解析:[第 159 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/387)
<br/>
第 158 题:如何模拟实现 Array.prototype.splice
解析:[第 158 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/384)
<br/>
第 157 题:浏览器缓存 ETag 里的值是怎么生成的
解析:[第 157 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/383)
<br/>
第 156 题:求最终 left、right 的宽度
```js
<div class="container">
<div class="left"></div>
<div class="right"></div>
</div>
<style>
* {
padding: 0;
margin: 0;
}
.container {
width: 600px;
height: 300px;
display: flex;
}
.left {
flex: 1 2 300px;
background: red;
}
.right {
flex: 2 1 200px;
background: blue;
}
</style>
```
注:此题和 155 题 left、right 样式有些不同
解析:[第 156 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/382)
<br/>
第 155 题:求最终 left、right 的宽度
```js
<div class="container">
<div class="left"></div>
<div class="right"></div>
</div>
<style>
* {
padding: 0;
margin: 0;
}
.container {
width: 600px;
height: 300px;
display: flex;
}
.left {
flex: 1 2 500px;
background: red;
}
.right {
flex: 2 1 400px;
background: blue;
}
</style>
```
解析:[第 155 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/381)
<br/>
第 154 题:弹性盒子中 flex: 0 1 auto 表示什么意思
解析:[第 154 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/380)
<br/>
第 153 题:实现一个批量请求函数 multiRequest(urls, maxNum)
要求如下:
1. 要求最大并发数 maxNum
2. 每当有一个请求返回,就留下一个空位,可以增加新的请求
3. 所有请求完成后,结果按照 urls 里面的顺序依次打出
解析:[第 153 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/378)
<br/>
2019-12-31
> 第 152 题:实现一个 normalize 函数,能将输入的特定的字符串转化为特定的结构化数据
解析:[第 152 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/331)
<br/>
2019-11-25
> 第 151 题:用最简洁代码实现 indexOf 方法
>
解析:[第 151 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/321)
<br/>
2019-11-21
> 第 150 题:二分查找如何定位左边界和右边界
>
> 不使用JS数组API,查找有序数列最先出现的位置和最后出现的位置
解析:[第 150 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/320)
<br/>
2019-11-12
> 第 149 题:babel 怎么把字符串解析成 AST,是怎么进行词法/语法分析的?
解析:[第 149 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/315)
<br/>
2019-11-01
> 第 148 题: webpack 中 loader 和 plugin 的区别是什么(平安)
解析:[第 148 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/308)
<br/>
2019-10-31
> 第 147 题:v-if、v-show、v-html 的原理是什么,它是如何封装的?
解析:[第 147 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/307)
<br/>
2019-10-29
> 第 146 题:Vue 中的 computed 和 watch 的区别在哪里(虾皮)
解析:[第 146 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/304)
<br/>
2019-10-24
> 第 145 题:前端项目如何找出性能瓶颈(阿里)
解析:[第 145 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/300)
<br/>
2019-10-22
> 第 144 题:手写二进制转 Base64(阿里)
解析:[第 144 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/299)
<br/>
2019-10-21
> 第 143 题:将 '10000000000' 形式的字符串,以每 3 位进行分隔展示 '10.000.000.000'
解析:[第 143 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/296)
<br/>
2019-10-17
> 第 142 题:(算法题)求多个数组之间的交集(阿里)
解析:[第 142 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/293)
<br/>
2019-10-15
> 第 141 题:Vue 中的 computed 是如何实现的(腾讯、平安)
解析:[第 141 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/291)
<br/>
2019-10-14
> 第 140 题:为什么 HTTP1.1 不能实现多路复用(腾讯)
解析:[第 140 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/290)
<br/>
2019-09-17
> 第 139 题:谈一谈 nextTick 的原理
解析:[第 139 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/281)
<br/>
2019-09-11
> 第 138 题:反转链表,每 k 个节点反转一次,不足 k 就保持原有顺序(哔哩哔哩)
解析:[第 138 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/278)
<br/>
2019-09-04
> 第 137 题:如何在 H5 和小程序项目中计算白屏时间和首屏时间,说说你的思路
解析:[第 137 题](https://github.com/Advanced-Frontend/Daily-Interview-Question/issues/272)
<br/>
## 所有面试题汇总
- [壹题所有题目及答案汇总](https://github.com/Advanced-Frontend/Daily-Interview-Question/blob/master/datum/summary.md)
<br/>
## 半月刊
- [前端 100 问:能搞懂 80% 的请把简历给我](https://github.com/yygmind/blog/issues/43)
- [【半月刊 1】前端高频面试题及答案汇总](https://juejin.im/post/5c6977e46fb9a049fd1063dc)
- [【半月刊 2】前端高频面试题及答案汇总](https://juejin.im/post/5c7bd72ef265da2de80f7f17)
- [【半月刊 3】前端高频面试题及答案汇总](https://juejin.im/post/5c9ac3f66fb9a070e056718f)
- [【半月刊 4】前端高频面试题及答案汇总](https://juejin.im/post/5cb3376bf265da039c0543da)
<br/>
## 联系我
进阶系列文章汇总如下,觉得不错点个 star,欢迎 **加群** 互相学习。
> [https://github.com/yygmind/blog](https://github.com/yygmind/blog)
我是木易杨,公众号「高级前端进阶」作者,跟着我**每周重点攻克一个前端面试重难点**。接下来让我带你走进高级前端的世界,在进阶的路上,共勉!
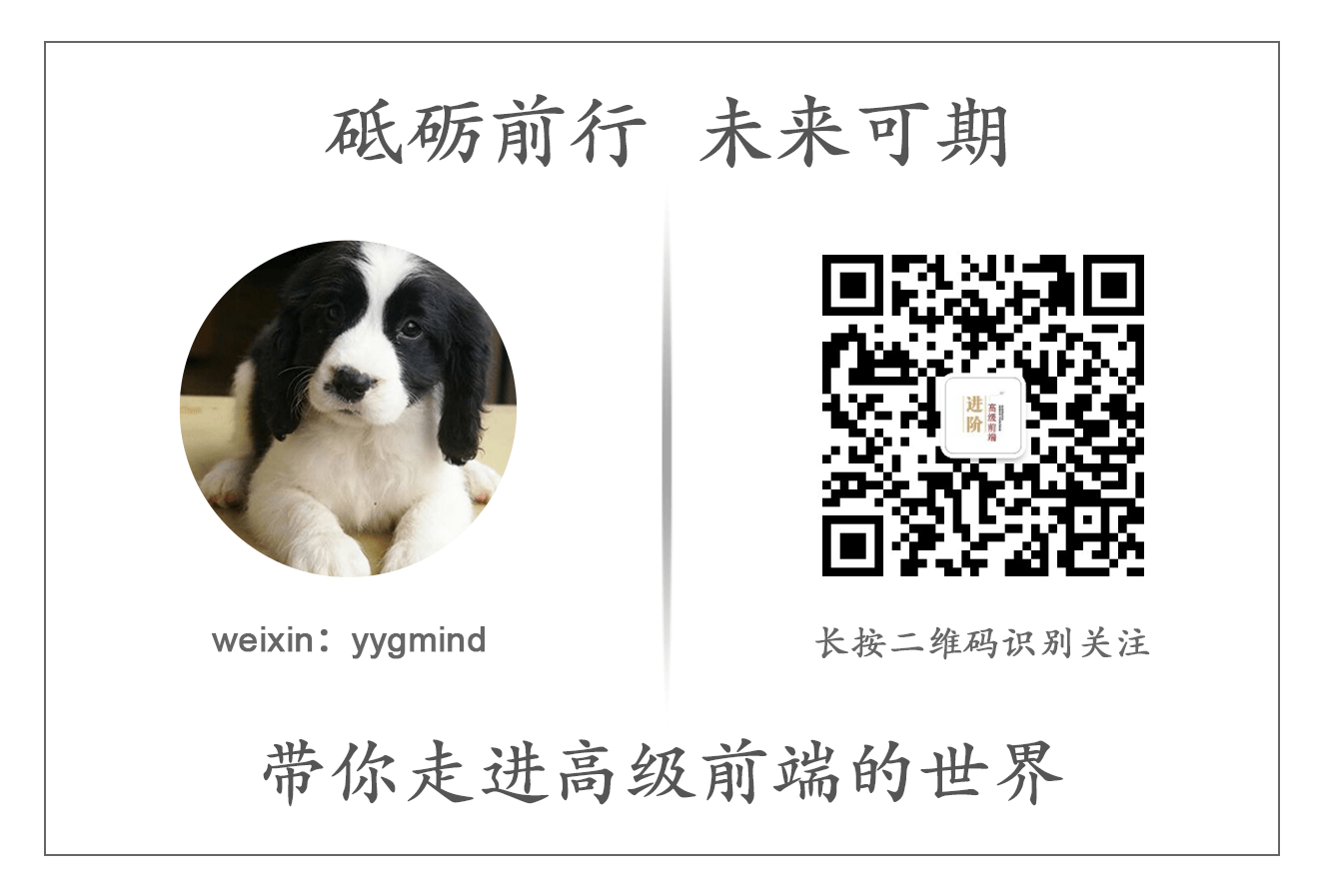